This post is part four of the No-Code 101 series, a straightforward guide to help non-technical professionals build powerful automations without writing code.
APIs are waiters
You're sitting in a restaurant. You want food, but you can't just walk into the kitchen and grab what you want. Instead, you talk to a waiter who takes your order to the kitchen and brings back your food.
APIs (Application Programming Interfaces) work just like that. Let’s say when you want to create a no-code automation that sends you a Slack notification every time you receive a new email from a specific email address:
- You = Your first app in the workflow (Gmail)
- The Kitchen = The next app in the workflow (Slack)
- The Waiter = The API
When your n8n workflow needs to get data from or send data to another application, it doesn't access that application directly. Instead, it talks to the application's API—the digital waiter that handles all communication between different software systems.
This is why APIs are so important: they're the reason your automation can talk to dozens of different applications without needing to understand how each one works internally.
HTTP: The language of APIs
Just like restaurants have standard ways to take orders ("How would you like that cooked?"), APIs use a standard language called HTTP (Hypertext Transfer Protocol).
Every HTTP request has these key components:
URL (The Address)
This determines where to send the request. For any application you want to connect with via an API, the API Reference will usually tell you. We call these URLs endpoints because you can imagine your software being a nerve cell and the APIs as the dendrites.
https://api.example.com/users
Method (The Action)
This will determine what you can actually do. Some endpoints are GET endpoints which means that they only accept HTTP requests (also known as API calls) with a GET method. Some accept multiple. You will always find that in the API reference.
- GET: Retrieve information (like reading your emails)
- POST: Create something new (like sending a tweet)
- PUT/PATCH: Update something (like changing a customer's address)
- DELETE: Remove something (like deleting a task)
Headers (The Special Instructions)
Extra information about your request. Think of it like an extra note to your order. Let’s say you have a coupon for a free meal at the restaurant so you will want to pass that on to the waiter as a special instruction showing that you’re authorized to eat there for free.
In most cases you’ll only deal with two types of Headers: Authorization
for API keys and Content-Type
which will be application/json
most of the time.
Authorization: Bearer abc123token
Content-Type: application/json
Body (The Order Details)
The data you're sending (only for POST, PUT, PATCH). You don’t send a Body with a GET request, because you’re requesting data not sending it. This is always used in JSON. If you don’t know what JSON is, read the previous lesson: Data Structures.
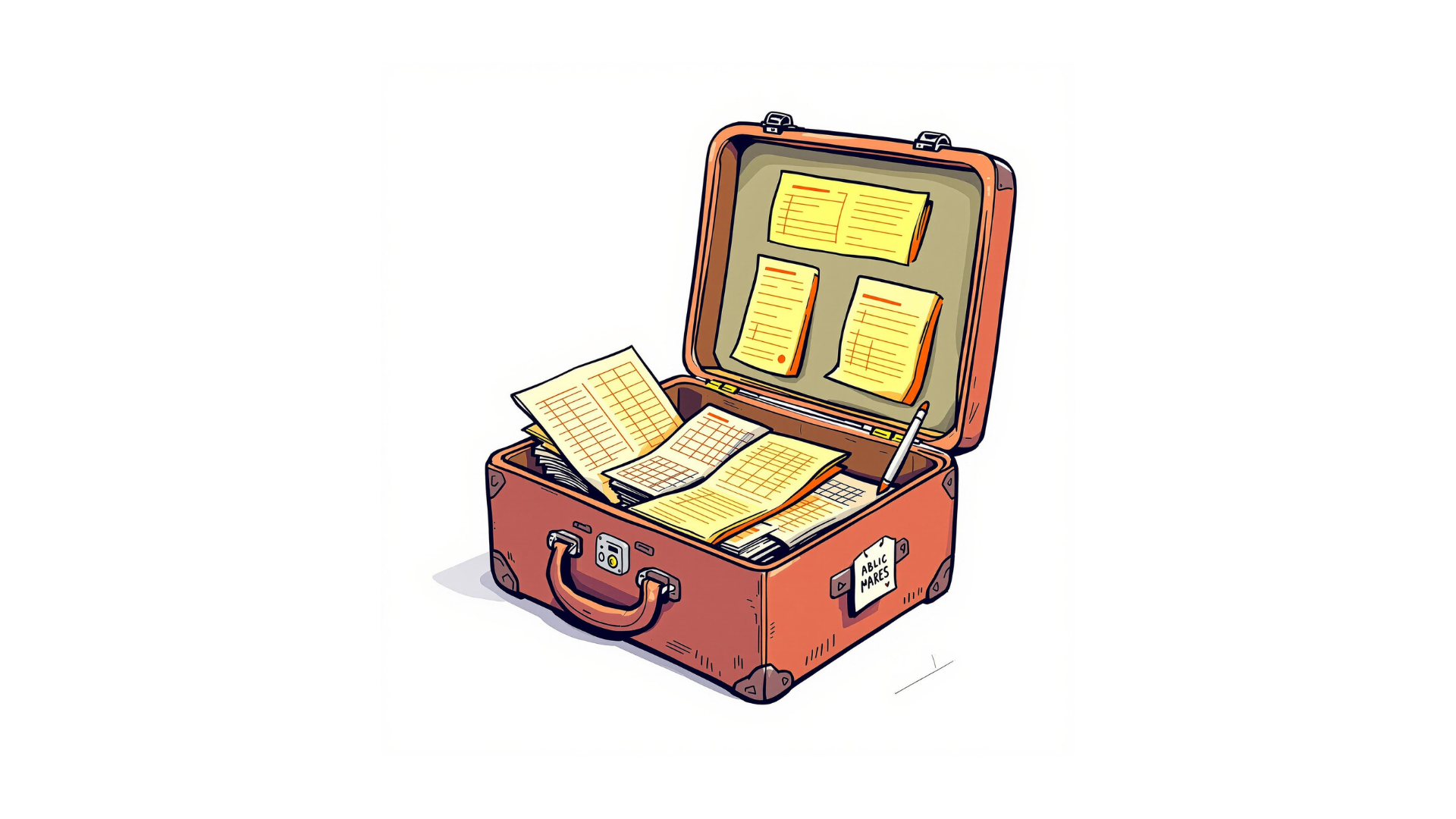
{
"name": "Alex Kim",
"email": "alex@example.com"
}
Parameters (The Special Requests)
Additional options or filters. This is a different kind of special request. This is not about whether or not you’re authorized but instead let’s say you’re lactose intolerant so you want to pass that along as a constraint. This can be a parameter. In most cases in n8n you won’t need to deal with this.
https://api.example.com/users?status=active&limit=10
API Authentication: The VIP Pass
Most APIs don't let just anyone access their data. They need to verify who you are first—that's authentication.
There are several common types:
1. API Keys
Like a simple password. You get a special string of characters and include it with every request as a parameter.
https://api.example.com/data?apikey=abc123xyz789
2. OAuth
Like having a valet ticket. Instead of giving your car keys (password) to every parking attendant, you get a special ticket (token) that proves you're authorized.
This is what happens when you click "Sign in with Google" and grant an app permission to access certain parts of your account.
If you’re using n8n in a self-hosted way say via Railway, you’ll need to set this up for yourself for every integration that uses OAuth. This includes most big tech platforms like Google or Microsoft.
If you want to learn how you can self-host n8n for almost free, check this:
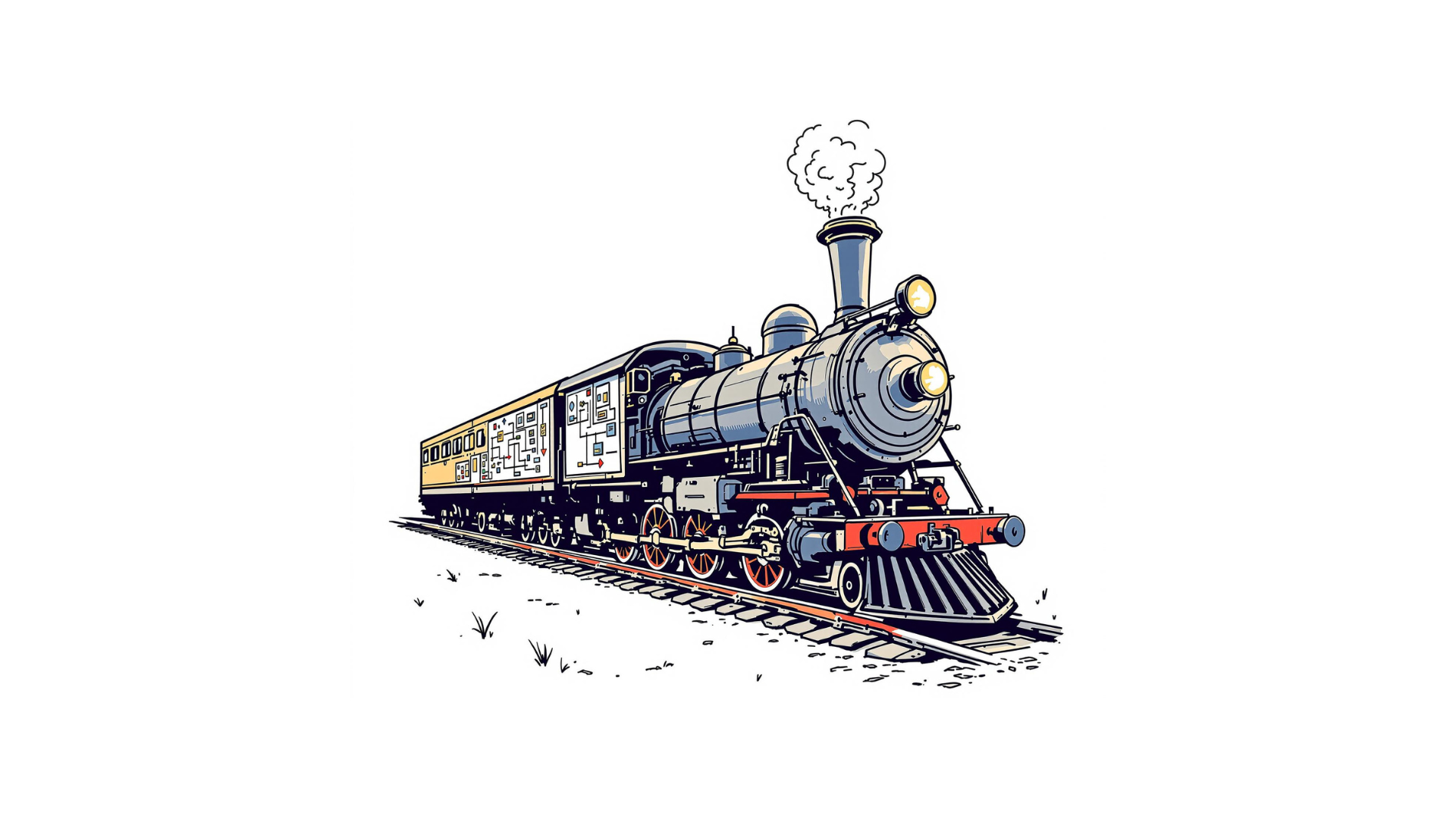
3. Basic Auth
Like giving your membership card at the entrance. You provide your username and password encoded in a special format. If the API key is defined as a “Bearer token” in the API reference, you’ll need to add “Bearer” before you paste in your API key.
Authorization: Bearer dXNlcm5hbWU6cGFzc3dvcmQ=
IMPORTANT: Whoever gets access to your API key can access your data. Never store your API keys in ways that’s easily accessible by others.
Using APIs in n8n: The Practical Part
In n8n, using APIs is remarkably simple thanks to the HTTP Request
node.
Let's walk through a real example:
Example: Getting Weather Data
Let's say you want to send your team a daily weather report in Slack. Here's how you would use an API for that:
Set up your workflow trigger (Time trigger for daily execution)
Add an HTTP Request node
- Set Method to GET
- URL:
https://api.weatherapi.com/v1/forecast.json?key=YOUR_API_KEY&q=London&days=1
- Authentication: None (API key is in URL parameters)
Add Code node to extract relevant data
Once our API call returned a bunch of weather data for us, we need to structure this in a nicer way. So we tell Claude/ChatGPT the following:
I have a JSON that I got from the Weather API. I want you to write me a JS script that returns some of the data in the following way:
Location: City (like London),
Temperature: Celsius (like 9)
Condition: Weather condition (like Light rain)
Forecast: Forecast (like Patchy rain nearby)
Here's the JSON:
{paste the JSON you got from your HTTP request node in n8n while testing}
Only return the code that I need to copypaste, no extra characters or no extra examples.
Then you get a code snippet that you just copy/paste into n8n:
const weatherData = {
location: $input.item.json.location.name,
temperature: $input.item.json.current.temp_c,
condition: $input.item.json.current.condition.text,
forecast: $input.item.json.forecast.forecastday[0].day.condition.text
};
// The Code node must return items array
return {
items: [{
json: weatherData
}]
};
Add Slack node to send message
As a last step you will need to add your Slack node with the action “Send a message”.
Your workflow will look something like this:

JSON❤️API
APIs love JSON. Most modern APIs send and receive data in JSON format.
When you make an API request, you'll typically get back a JSON response like:
{
"location": {
"name": "London",
"country": "UK"
},
"current": {
"temp_c": 18,
"condition": {
"text": "Partly cloudy"
}
}
}
In n8n, this becomes available as $json
in subsequent nodes. For example, to access the temperature, you would use {{$json.current.temp_c}}
. If you need to specifically refer to a node in a workflow, then you can do that like this: {{$node[‘Node Name’].json.current_temp_c}}
Postman: Your API Testing Ground
Before building your workflow, it's often helpful to test API requests. Postman is a free tool perfect for this:
- Download Postman
- Create a new request
- Enter your URL, method, headers, and body
- Hit Send to see the response
This helps you understand what data you'll get back before building your n8n workflow.
Common API Challenges & Solutions
1. Rate Limits
Most APIs limit how many requests you can make (e.g., 1000 per day).
Solution: Add delay nodes between requests or use n8n's built-in retry logic.
2. Authentication Errors
The dreaded "401 Unauthorized" error.
Solution: Double-check your API key, token expiration, or credentials.
Copy
If using OAuth, check if your token has expired. Tokens typically last 1-2 hours.
3. Data Format Issues
API expects one format, but you're sending another.
Solution: Always check the Content-Type
header and ensure your data structure matches the API documentation.
4. Finding the Right Endpoint
Not sure which URL to use for what you need.
Solution: Read the API documentation. Most provide a list of all available endpoints and what they do.
Using AI to Master APIs
Let's say you want to integrate with a new service but aren't sure how their API works. Here's how AI can help:
Ask for API basics:
I want to integrate with [Service Name]'s API in n8n. Can you explain how to authenticate and what the main endpoints are?
Generate HTTP Request configurations:
I need to [what you want to do] with [Service Name]. Can you show me the HTTP Request configuration I'd need in n8n?
Troubleshoot errors:
I'm getting this error from [Service Name]'s API: [paste error]. What might be causing it?
I'm getting this error from [Service Name]'s API: [paste error]. What might be causing it?
LLMs are excellent at helping you understand documentation and troubleshoot issues—often faster than combing through docs yourself! Just make sure that if it makes errors or can’t solve your problem, paste the API reference to it so it knows what to work with.
TL;DR for Non-Developers
APIs = Digital waiters that help applications talk to each other
HTTP Methods:
GET = Read data
POST = Create data
PUT/PATCH = Update data
DELETE = Remove data
Authentication = Your VIP pass to access the API
n8n's HTTP Request node = Your universal connector to any API
Postman = Test APIs before building workflows
AI = Your API assistant for documentation and troubleshooting
Model Context Protocol: Next gen API
While APIs connect different software systems, a newer protocol called Model Context Protocol (MCP) is emerging specifically for AI interactions. Recently open-sourced by Anthropic, MCP represents a significant advancement in how AI systems access and maintain context.
What is MCP?
Think of MCP as a specialized bridge designed specifically for AI systems to connect with data sources:
- APIs are like general-purpose highways connecting software applications
- MCP is like a dedicated neural pathway connecting AI models directly to data sources
The fundamental difference is in how they handle context:
- APIs typically handle single, stateless transactions: "Here's some data, do something with it, give me a response"
- MCP maintains ongoing contextual awareness: "Here's access to my database, remember what you find, and use it throughout our conversation"
Why MCP Matters for you
As you build more sophisticated automations with AI components, MCP could solve several key challenges:
- Data Access Without Copying: Rather than copying data via API and sending it to an AI, MCP gives the AI direct, secure access to where the data lives.
- Context Persistence: MCP helps AI maintain awareness of information across multiple interactions, eliminating the need to repeatedly provide the same context.
- Unified Connection Standard: Instead of creating custom integrations for every data source, MCP provides a standard protocol—potentially simplifying how you connect AI with your business tools.
How MCP Works (Simplified)
MCP establishes a two-way connection between:
- MCP Servers: These expose your data sources (like Google Drive, Slack, or GitHub)
- MCP Clients: AI applications that connect to these servers
For example, instead of writing code or setting up a node in n8n to extract data from your CRM, format it, and send it to an AI through an API, you could set up an MCP server for your CRM. The AI could then directly query relevant information as needed throughout a conversation.
The promise is that you should be able to generate something like a n8n workflow on the fly without having to determine everything personally.
While still in its early stages, MCP represents an important step in how we'll integrate AI into our automation workflows. For no-code builders, this means future tools may offer simpler ways to create context-aware AI agents that can reason across your business systems without the complexity of managing multiple API connections.
As n8n and other no-code platforms evolve, watch for MCP integration options that might make building sophisticated AI workflows more accessible to non-technical builders. I’m also going to do a deep dive into how MCP works in the coming days/weeks.
That’s it. This concludes the four part introduction series into no-code. Once you understand these concepts you understand everything you need in order to build n8n workflows. Now all you need to do is practice, practice, practice!